Image Processing based on OpenCv with Python on Raspbian, Raspberry Pi
First Steps with Image processing based on Rasberry Pi, OpenCV & Python 3.6.1 on Debian Jessie
Python environment, libraries
Contents
Update Python 2.7
update setup tools
sudo apt-get install python-setuptools
install packets tool
sudo easy_install pip
How to install Python 3.6.1 on Raspbian Jessie
sudo echo 'deb http://mirrordirector.raspbian.org/raspbian/ testing main contrib non-free rpi' > /etc/apt/sources.list.d/stretch.list
sudo apt-get update
sudo apt-get dist-upgrade
sudo apt-get autoremove
First of all you need to get your dependencies right. That mostly depend on what you have already installed previously. So for a vanilla fresh Raspbian (jessie), you will (approximately) need to make sure you have these:
sudo apt-get install build-essential libc6-dev
sudo apt-get install libncurses5-dev libncursesw5-dev libreadline6-dev
sudo apt-get install libdb5.3-dev libgdbm-dev libsqlite3-dev libssl-dev
sudo apt-get install libbz2-dev libexpat1-dev liblzma-dev zlib1g-dev
The rest is simple. First download and extract…
cd $HOME
wget https://www.python.org/ftp/python/3.6.1/Python-3.6.1.tgz
tar -zxvf Python-3.6.1.tgz
compile with
cd Python-3.6.1 ./configure --enable-optimizations
We have 4 processors, so let’s use 4 threads or
make -j4
in RPI ZERO 1
make
sudo make altinstall
Save your SD card space
cd ..
sudo rm -fr ./Python-3.6.1*
Now test with
cd
python3.6 -V
# "Python 3.6.1"
pip3.6 list
Output
pip (9.0.1) setuptools (28.8.0)
if is necessary to install pip , a Python package manager
sudo pip3.6 install --upgrade pip
sudo pip3.6 install -U pip
sudo pip3.6 install -U setuptools
Alternative for Install Python 3.6.1
http://www.pyimagesearch.com/2016/04/18/install-guide-raspberry-pi-3-raspbian-jessie-opencv-3/
A. with packages, shorter process on small device RPI ZERO W
sudo nano etc/apt/sources.list
# add
deb http://ftp.de.debian.org/debian experimental main
deb http://ftp.de.debian.org/debian unstable main
deb http://http.debian.net/debian/ jessie main contrib non-free
sudo apt-get update
sudo apt-get install python3.6
python3.6 -V
Install with Python 2.7
Install Raspberry Camera, 8MP
sudo pip3.6 install picamera
Before Install OpenCv
Installing Build Dependencies
To compile OpenCV, we must ensure that the required dependencies are available, including the build tools themselves. We can get the required ones using apt
on Ubuntu, but first, ensure the package list is up-to-date by running apt-get update
. Next, execute the following commands to get the required packages (see below for a one-line list of all):
sudo apt-get install cmake build-essential pkg-config
sudo apt-get install libgtk2.0-dev libtbb-dev
sudo apt-get install python-dev python-numpy python-scipy
sudo apt-get install libjasper-dev libjpeg-dev libpng-dev libtiff-dev
sudo apt-get install libavcodec-dev libavutil-dev libavformat-dev libswscale-dev
sudo apt-get install libdc1394-22-dev libv4l-dev
In single line
sudo apt-get install cmake build-essential pkg-config libgtk2.0-dev libtbb-dev python-dev python-numpy python-scipy libjasper-dev libjpeg-dev libpng-dev libtiff-dev libavcodec-dev libavutil-dev libavformat-dev libswscale-dev libdc1394-22-dev libv4l-dev
Install libraries
sudo pip3.6 install numpy
Setup Python
The first step in setting up Python for our OpenCV compile is to install pip , a Python package manager:
/usr/local/bin/pip install virtualenv virtualenvwrapper # OR sudo pip3.6 install virtualenv virtualenvwrapper sudo rm -rf ~/.cache/pip3.6
mkvirtualenv cv # OR mkvirtualenv --python=python3.6 cv # OR mkvirtualenv cv -p python3.6
source ~/.profile workon cv python -V

on rpi ZERO
http://www.pyimagesearch.com/2015/12/14/installing-opencv-on-your-raspberry-pi-zero/
Camera Test
Steps to install PyGame using pip
-
- Install build dependencies (on linux):
sudo apt-get build-dep python-pygame
- Install mercurial to use
hg
(on linux):sudo apt-get install mercurial
- Use pip to install PyGame:
pip3.6 install hg+http://bitbucket.org/pygame/pygame
If the above gives
freetype-config: not found
error (on Linux), then trysudo apt-get install libfreetype6-dev
and then repeat 3.
- Install build dependencies (on linux):
Alternative way
sudo apt-get install python3-pygame
OR
python3.6 -m pip install pygame --user
python3.6 -m pygame.examples.aliens
Install on virtualenvironment
pip3.6 install numpy
Install OpenCv v2
from linux packages
sudo apt-get install python-opencv sudo apt-get install python-scipy sudo apt-get install ipython
from OpenCv repository
https://pypi.python.org/pypi/opencv-python/3.1.0.3
cd ~ wget -O opencv.zip https://github.com/Itseez/opencv/archive/3.1.0.zip unzip opencv.zip
We’ll want the full install of OpenCV 3 (to have access to features such as SIFT and SURF, for instance), so we also need to grab the opencv_contrib repository as well:
wget -O opencv_contrib.zip https://github.com/Itseez/opencv_contrib/archive/3.1.0.zip unzip opencv_contrib.zip
Compiling
cd ~/opencv-3.1.0/ mkdir build cd build cmake -D CMAKE_BUILD_TYPE=RELEASE -D CMAKE_INSTALL_PREFIX=/usr/local -D OPENCV_EXTRA_MODULES_PATH=../../../../opencv_contrib-3.1.0/modules -DBUILD_TIFF=ON -DBUILD_opencv_java=OFF -DWITH_CUDA=OFF -DENABLE_AVX=ON -DWITH_OPENGL=ON -DWITH_OPENCL=ON -DWITH_IPP=ON -DWITH_TBB=ON -DWITH_EIGEN=ON -DWITH_V4L=ON -DBUILD_TESTS=OFF -DBUILD_PERF_TESTS=OFF -DCMAKE_BUILD_TYPE=RELEASE
cmake -D CMAKE_BUILD_TYPE=RELEASE -D CMAKE_INSTALL_PREFIX="../../../../../../usr/local" -D OPENCV_EXTRA_MODULES_PATH=../../../../opencv_contrib-3.1.0/modules -DBUILD_TIFF=ON -DBUILD_opencv_java=OFF -DWITH_CUDA=OFF -DENABLE_AVX=ON -DWITH_OPENGL=ON -DWITH_OPENCL=ON -DWITH_IPP=ON -DWITH_TBB=ON -DWITH_EIGEN=ON -DWITH_V4L=ON -DBUILD_TESTS=OFF -DBUILD_PERF_TESTS=OFF -DCMAKE_BUILD_TYPE=RELEASE
makeing
make clean make sudo make install sudo ldconfig
Verifying Installation
As mentioned previously, you can verify that the cv2
module was installed correctly by executing the following in a Python shell:
import cv2
print(cv2.__version__)
Image Processing
More info
Rpi Camera Documentation
http://picamera.readthedocs.io/en/release-1.10/api_camera.html
Rpi Camera Start
http://www.netzmafia.de/skripten/hardware/RasPi/RasPi_Kamera.html
Pi Camera Calibration (image undistortion)
Pi Camera with GoPro Lens Distortion Removal (for Stream and Video undistortion)
https://www.theeminentcodfish.com/gopro-calibration/
Pi Camera with OpenCv Text detection
https://github.com/opencv/opencv_contrib/blob/master/modules/text/samples/textdetection.py
About OpenCv

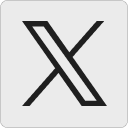



